Automated testing has become indispensable for ensuring the reliability and performance of web applications. End-to-end testing frameworks like Playwright have emerged as powerful tools enabling developers and testers to simulate real user interactions across browsers. A fundamental aspect of effectively using Playwright is mastering Playwright Locators—tools that allow precise identification and manipulation of web elements.
This detailed guide aims to comprehensively understand Playwright Locators, their types, usage, and best practices to enhance your automation testing strategies.
What is Playwright?
Playwright is an open-source Node.js library developed by Microsoft to automate web browsers. It provides a high-level API to control Chromium and WebKit, enabling cross-browser testing with a single codebase. Playwright is designed to be reliable, fast, and capable of handling modern web applications that heavily rely on JavaScript.
Key Features of Playwright
- Cross-Browser Support: Automate tests across Chromium, Firefox, and WebKit.
- Auto-Wait Mechanism: Automatically waits for elements to be ready before performing actions.
- Isolation Model: Offers browser contexts that isolate cookies and cache, making parallel testing more efficient.
- Network Control: Intercept and modify network requests and responses.
- Extensive API: Provides a rich set of functionalities for various testing needs.
Installing and Setting Up Playwright
Prerequisites
- Node.js: Ensure you have Node.js (version 14 or higher) installed.
- Package Manager: npm or Yarn.
Project Initialization
Browser Installation
Getting Started with Playwright
Writing Your First Test
Let's write a simple test to ensure the Playwright website's title contains "Playwright".
Step 1: Create a Test File
Create a new file named example.spec.ts
inside the tests directory:
touch tests/example.spec.ts
Step 2: Write the Test Code
Open example.spec.ts
in your code editor and add the following code:
Step 3: Add Another Test (Optional)
Running the Tests
The test runner will search for files matching *.spec.ts
or *.spec.js
in the tests
directory and execute them.
Expected Output
This indicates that both tests have passed successfully.
Also read: Know All About Cross Browser Compatibility Testing
Understanding Playwright Locators
What Are Locators?
Locators in Playwright are objects used to find elements on a web page. They are the cornerstone for interacting with web elements—clicking buttons, entering text, selecting options, and more. By using locators, you can write scripts that mimic real user actions, essential for thorough end-to-end testing.
Purpose of Locators
- Element Identification: Precisely target elements for interaction.
- Automation: Simulate user behavior in a consistent and repeatable manner.
- Verification: Assert the state and properties of elements during tests.
- Maintainability: Write resilient tests that withstand changes in the application's UI.
Locators vs. Selectors
- Selectors: Strings that describe locating elements in the DOM, such as CSS selectors or XPath expressions.
- Locators: Abstractions built on selectors that offer additional methods for interaction and assertions. They provide a more robust and flexible way to work with elements.
When to Use Locators vs. Selectors
Drawbacks of Using Selectors Directly vs. Advantages of Using Locators
Importance in Testing
Using locators effectively is crucial for the following:
Test Reliability
Test reliability reduces false negatives caused by flaky tests. Precise and robust locators ensure that your test scripts interact with the correct web elements every time. By accurately identifying elements, you reduce the risk of tests failing due to elements not being found or incorrect elements being manipulated.
Efficiency
Locator efficiency minimizes the time spent writing and maintaining tests. Effective locators simplify your test scripts, making them more readable and easier to maintain. Using straightforward and consistent locator strategies reduces the time spent debugging and updating tests. When the application's UI changes, well-crafted locators are easier to adjust.
Scalability
Locator scalability allows tests to grow with the application without becoming unmanageable. As your application grows, the number of elements and interactions increases. Using modular and reusable locator strategies allows your tests to scale alongside your application without becoming unwieldy.
Overview of Locator Syntax
Types of Playwright Locators
Playwright offers a variety of locators to suit different test scenarios. Understanding these helps in selecting the most effective locator for each element.
Label locators
The getByLabel
locator allows you to find elements that contain a specific text string. It searches through the page's rendered text content and matches elements whose visible text corresponds to the provided value.
Placeholder locators
The getByPlaceholder
locator finds input elements using their placeholder attribute value. The placeholder
text is the hint or example text displayed inside input fields when empty.
AltText locators
The getByAltText
locator finds elements with an alt
attribute matching the specified text, commonly used with images (<img>
tags). The alt
attribute provides alternative information for an image if a user cannot view it and is a key component of web accessibility.
Title locators
The getByTitle
locator allows you to find elements by their title
attribute value. The title
attribute is often used to provide additional information about an element, typically displayed as a tooltip when the user hovers over it.
Test ID locators
The getByTestId
locator is designed to find elements using a data attribute specifically for testing purposes, such as data-testid
. Using a dedicated attribute for test selection, you decouple your tests from the styling and structure of the application, making them more resilient to changes in the UI.
Text Locators
Matching by text helps target elements with their visible text content. It is useful to find elements containing specific text that are useful for buttons or links.
Role Locators
Select elements based on their ARIA roles, improving accessibility testing. Role selectors allow tests to interact with elements in the same way assistive technologies interpret and engage with web content.
CSS Selectors
CSS selectors help select elements in browsers effectively and efficiently. Utilize standard CSS syntax to select elements based on classes, IDs, attributes, and hierarchical relationships.
XPath Selectors
XPath selectors help select elements based on their HTML content and structure. Leverage XPath Path language to navigate the DOM tree.
Nth Selectors
Target elements based on their position in a list or hierarchy.
React Selectors
For applications built with React, Playwright provides special selectors to locate components by their name or props, facilitating testing of React-specific components.
Note: React selectors rely on internal knowledge of the application's component hierarchy and require the application to be in development mode. They are particularly useful when you can access the component's source code and need to test components in isolation.
Vue Selectors
Similarly, for Vue.js applications, Playwright offers selectors the ability to locate Vue components by name or props.
Note: Vue selectors require the application to expose component details and are best used in development environments. They allow for more granular testing of Vue components, especially when you need to interact with component-specific properties or methods.
Best Practices for Effective Locator Usage
Leverage Playwright's Built-in Locators
Playwright's locators come with auto-waiting and retry mechanisms, ensuring actions are performed on ready elements.
Prefer User-Facing Attributes to XPath or CSS Selectors
Relying on the DOM structure or CSS classes can lead to fragile tests that break when the UI changes. Instead, use locators based on user-facing attributes like roles and accessible names.
Leverage Auto-Waiting
Playwright automatically waits for elements to be actionable before performing actions on them. Utilize this feature to write cleaner code without explicit waits.
Use Data Attributes for Stable Selectors
Consider adding custom data-testid
attributes to your elements when built-in locators are insufficient. This provides a stable way to locate less likely to change elements.
Use Class Names Carefully
Class names can be used when IDs or accessibility IDs are not available. However, they may not be unique and can change if the UI is updated.
Considerations:
- Non-Unique: Multiple elements may share the same class.
- Maintenance: Changes in styles can affect selectors.
Utilize Native Locator Strategies When Necessary
Playwright supports native locator strategies interpreted by the underlying automation frameworks, such as -android uiautomator
, -ios predicate string
, and -ios class chain
.
Use Image Locators as a Last Resort
Image locators (-image
) allow you to find elements based on images. While useful in certain scenarios, they should be used sparingly.
Also check: How to Pick the Right Locator Strategy
Using Playwright with HeadSpin
To use Playwright with HeadSpin, first start a browser session.
Launch the Browser with HeadSpin CLI:
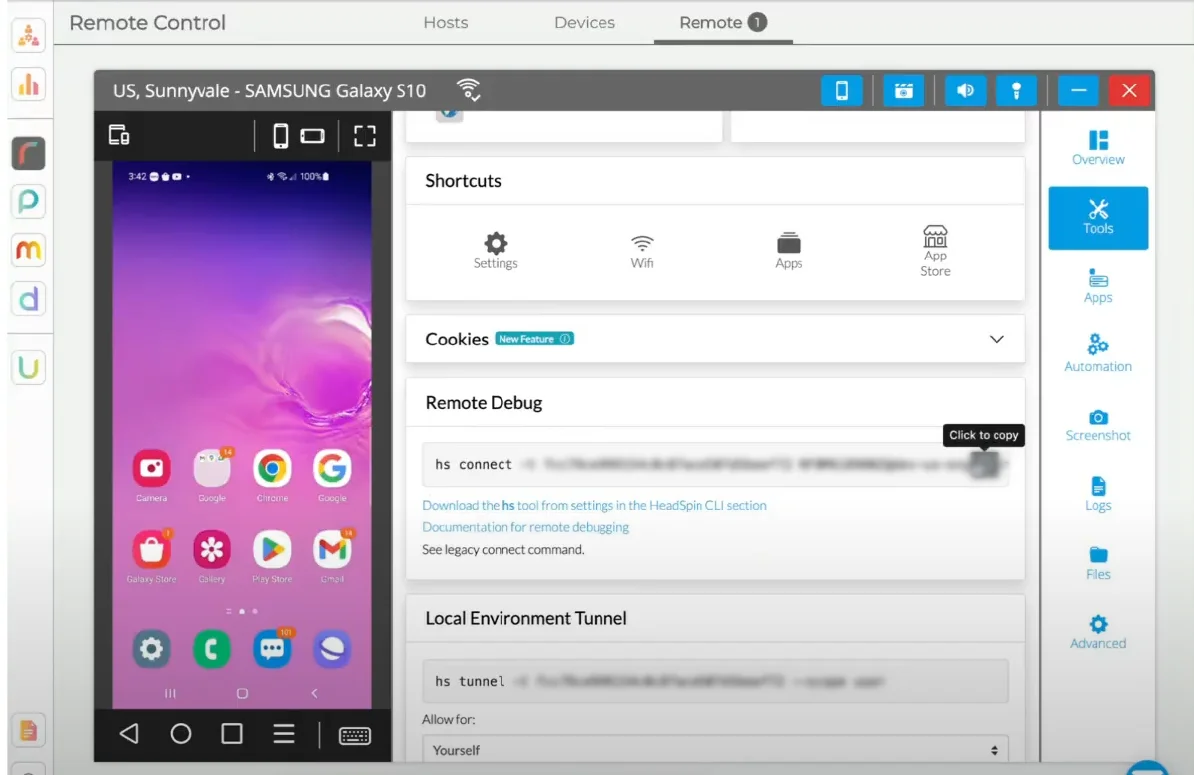
● Run the hs connect command:
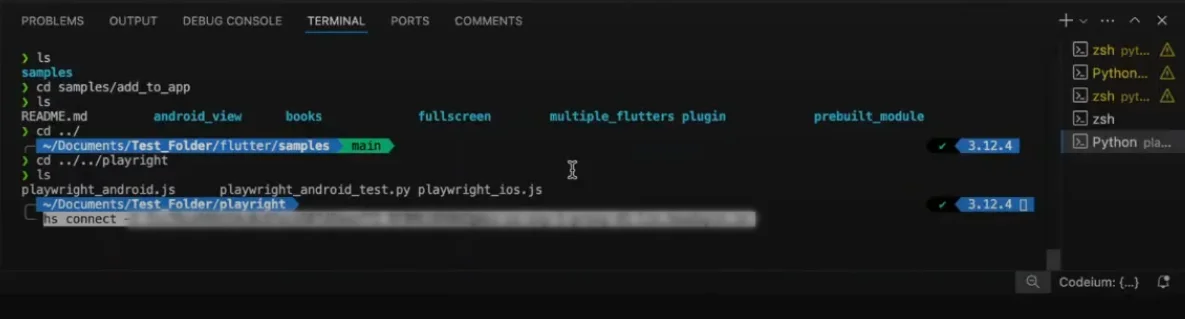
Sample piece of code that imports Playwright:
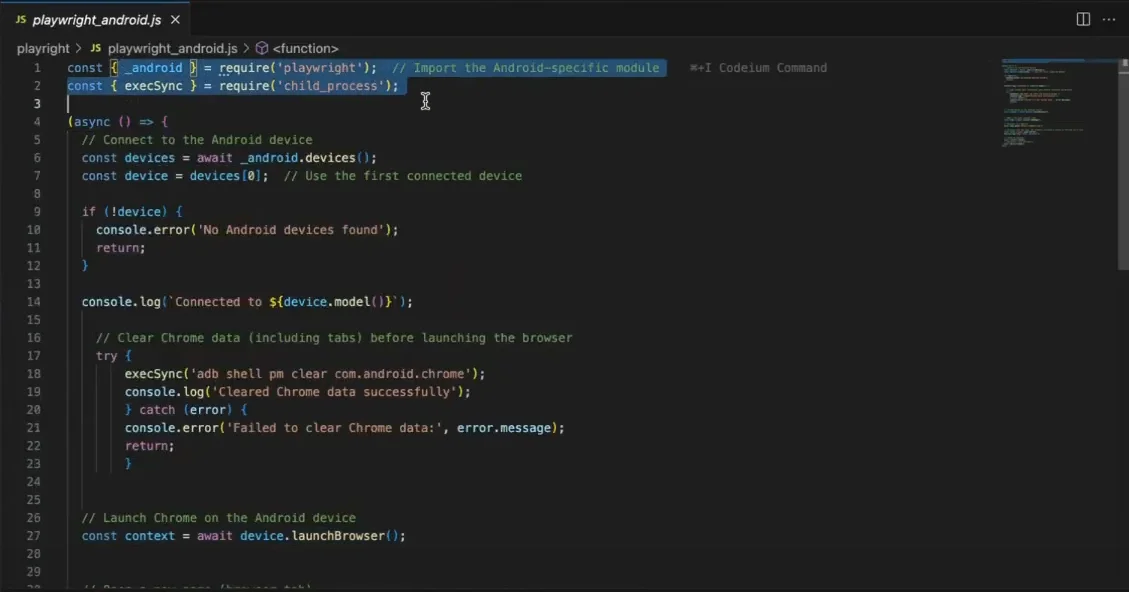
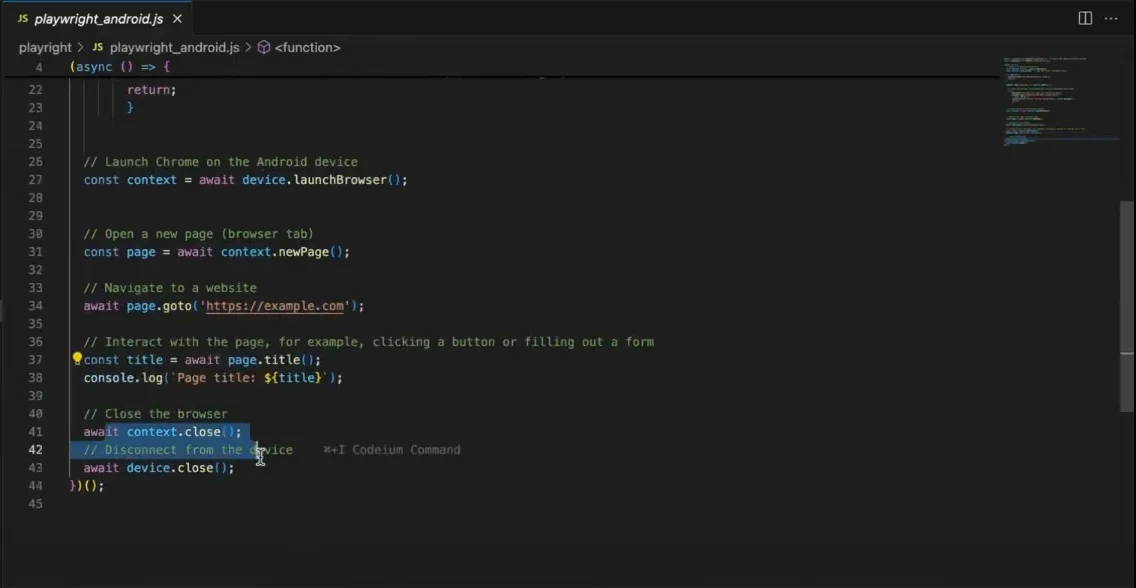
Run the Playwright Tests:
● This will run all the test cases your script defines against the browser on the mobile Android device that is hs connected.

● If it runs successfully, you will see the URL opening on the device.
● You can check the test on the HeadSpin Platform.
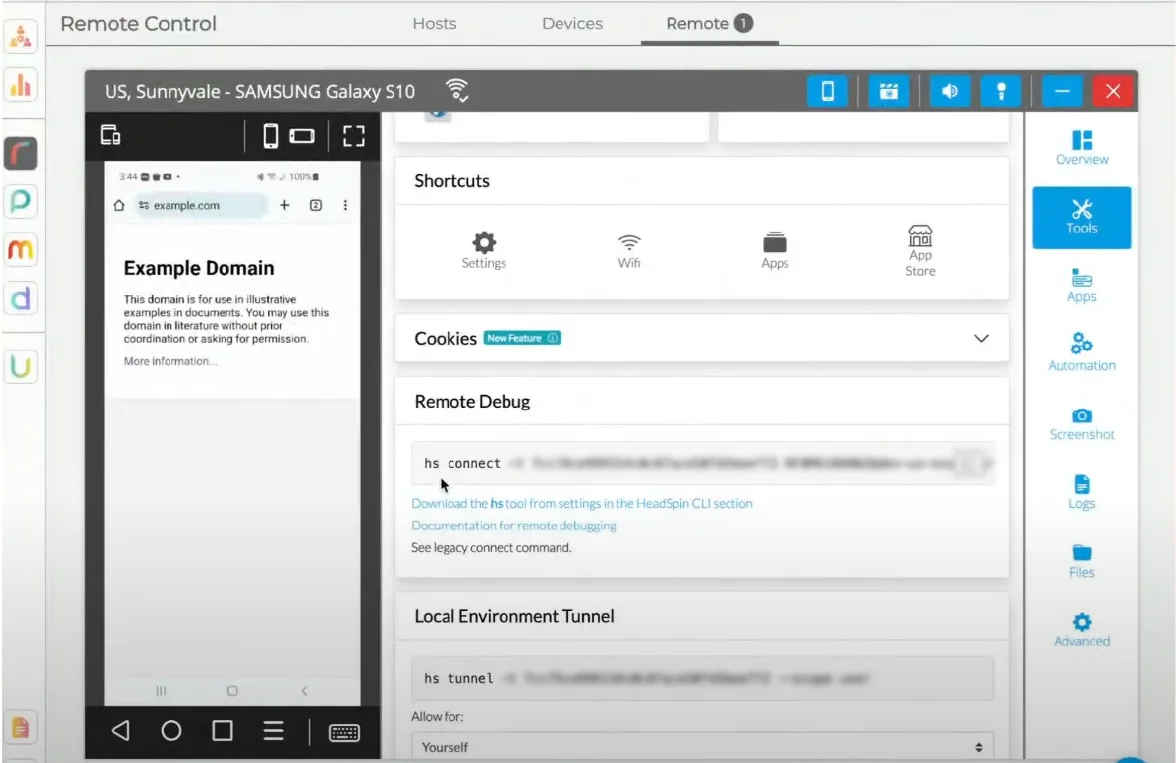
● The test will open a new tab and navigate to the specified webpage - in this case - example.com.
● In your terminal, you see how Playwright opens the webpage and grabs its title.
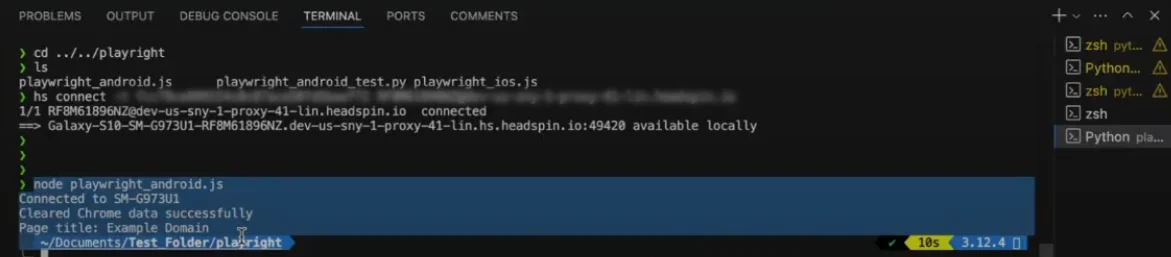
Enhancing Testing with HeadSpin Integration
While Playwright provides robust tools for automation, integrating it with a platform like HeadSpin can significantly boost your testing capabilities.
Use Cloud-Based Testing Environments
- Scalability: Run tests across multiple environments without local setup.
- Accessibility: Access a wide range of browsers and devices.
Run Tests in Parallel
- Efficiency: Reduce test execution time by running tests concurrently.
- Coverage: Test multiple scenarios simultaneously.
Monitor Test Performance and Results
- Analytics: Gain insights through detailed performance metrics.
- Reporting: Generate comprehensive test reports.
Integrate into CI/CD Pipelines
- Automation: Trigger tests automatically on code changes.
- Consistency: Ensure that every build is tested thoroughly.
Ensure Tests Are Maintainable and Scalable
- Modular Design: Write reusable code components.
- Version Control Integration: Keep track of changes and collaborate effectively.
Conclusion
Mastering Playwright Locators is a pivotal step in enhancing your end-to-end testing strategy. By understanding the various locator types and best practices, you can write tests that are reliable, maintainable, and reflective of real user interactions.
Integrating Playwright with advanced testing platforms like HeadSpin further amplifies your testing efficiency. The combination of robust locator strategies and powerful testing tools ensures that your web applications are thoroughly tested, delivering high-quality software to users.
FAQs
Q1. What are Playwright Locators, and why are they important?
Ans: Playwright Locators are tools for identifying and interacting with web elements during automated testing. They are essential because they allow for precise element targeting, making tests more reliable and reflective of user behavior.
Q2. How do CSS selectors differ from XPath selectors in Playwright?
Ans: CSS selectors use the Cascading Style Sheets syntax to select elements based on classes, IDs, and attributes, offering simplicity and performance. XPath selectors use XML path expressions to navigate the DOM, providing flexibility for complex structures. The choice depends on test case specific requirements.
Q3. Can I use Playwright to test applications with dynamic content?
Ans: Yes, Playwright is well-suited for testing dynamic applications. Its auto-wait features and the ability to wait for specific network states ensure that tests interact with elements only after they are fully loaded.
Q4. How can I make my Playwright tests more maintainable and less flaky?
Ans: To enhance maintainability and reduce flakiness:
- Use Stable Locators: Opt for unique selectors like IDs or data attributes.
- Implement Proper Waiting: Utilize Playwright's waiting mechanisms instead of hard-coded delays.
- Organize Code: Employ the Page Object Model to structure your tests.
- Keep Tests Independent: Ensure tests do not rely on the state left by previous tests.
- Regularly Update Tests: Keep your test scripts in sync with application changes.
Q5. What are the benefits of integrating Playwright tests with a platform like HeadSpin?
Ans: Integrating with HeadSpin offers:
- Cloud-Based Testing: Access to various browsers and devices without local setup.
- Parallel Execution: Run multiple tests simultaneously to save time.
- Detailed Analytics: In-depth performance metrics and reporting.
- CI/CD Integration: Seamless incorporation into development pipelines.
- Scalability: Easily scale your testing efforts as your application grows.